Due: Tuesday Feb 11, before 9:00 pm
Objectives
- Review the procedures to access the GL servers and to compile programs on GL.
- Review C++ memory management (allocating and deallocating memory dynamically).
- Use Valgrind to check for memory leaks.
- To practice writing unit tests.
- To practice working in a Linux environment.
- To practice analyzing and understanding a project requirements.
Introduction
In this project, you will complete a C++ class. Furthermore, you will write a tester class and a test program and use Valgrind to check that your program is free of memory leaks. Finally, you will submit your project files on GL. If you have submitted programs on GL using shared directories (instead of the submit command), then the submission steps should be familiar.
This application will hold the information about a College course which has multiple sections. The user of this application can perform statistical analysis on the grades at different levels such as a specific section or all sections together. For example, the user can calculate the average for grades for a specific exam in a section of class.
The application has two C++ classes, i.e. Section and Course. An object of the Section class stores the students’ grades in a 2D array. An object of the Course class stores pointers to the Section objects in an array. All arrays are created dynamically since the users need to analyze sections and classes of different sizes.
The following figures present a graphical realization of the main data structures in this application. The 2D array in the Section class is stored in the member variable m_section. The 2D array in the Course class is stored in the member variable m_course.
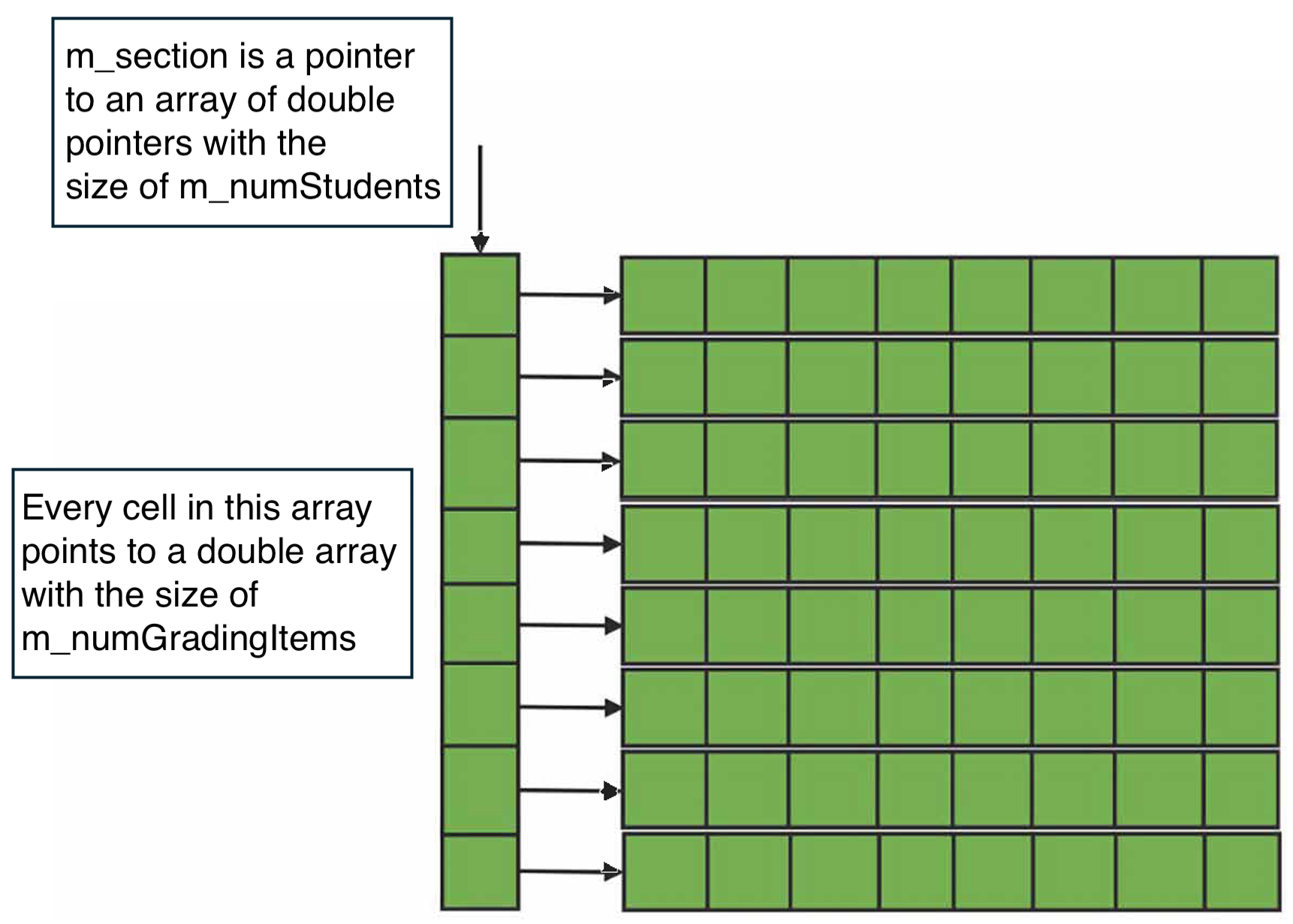
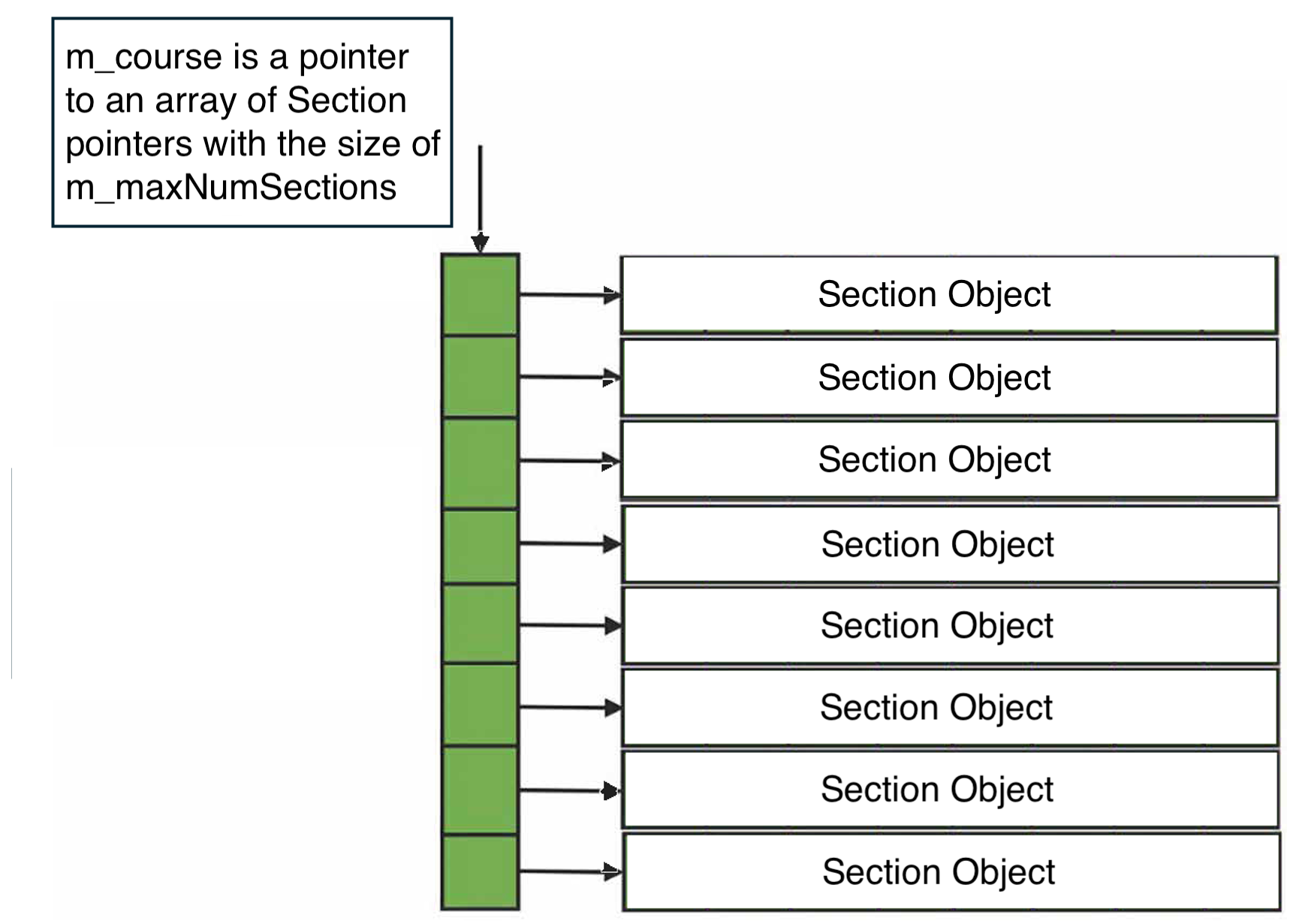
Assignment
Step 1: Create your working directory
Create a directory in your GL account to contain your project files. For example, cs341/proj0.
Step 2: Copy the project files
You can right-click on the file links on this page and save-as in your computer. Then, transfer the files to your GL account using SFTP.
For this project, you are provided with the skeleton .h and .cpp files, a sample driver, and the output of driver program:
- course.h - The interface for the classes Section and Course.
- course.cpp - The skeleton for the class implementation.
- driver.cpp - The sample driver/test program.
- driver.txt - The output of sample driver/test program.
Step 3: Complete the Section and Course classes
You need to implement the classes according to the requirements. For the requirements please refer to the Specifications, The Rules, and Additional Requirements sections on this page.
Step 4: Test your code
You need to test your implementation properly and adequately. For a description of testing please refer to the Testing section on this page. Moreover, a sample test function has been provided in the file driver.cpp for your reference.
Step 5: Check for memory leaks
Run your test program using Valgrind. For example, assuming you have compiled mytest.cpp, producing the executable mytest.out, run the command
valgrind mytest.out
If there are no memory leaks, the end of the output should be similar to the following:
==8613== ==8613== HEAP SUMMARY: ==8613== in use at exit: 0 bytes in 0 blocks ==8613== total heap usage: 14 allocs, 14 frees, 73,888 bytes allocated ==8613== ==8613== All heap blocks were freed -- no leaks are possible ==8613== ==8613== For lists of detected and suppressed errors, rerun with: -s ==8613== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
The important parts are “in use at exit: 0 bytes” and “no leaks are possible.” The last line is also important as memory errors can lead to leaks.
Step 6: Link your shared directory
Please take this step as soon as you receive the notification indicating the shared submission folders are ready.
Leaving this step to the last minute can prevent you from submitting your work on time.
Please note:
* No other method of submission will be accepted due to such a problem.
* No late submission will be accepted due to such a problem.
Follow the instructions on the Project Submission page to make a symbolic link to the shared directory in your home directory.
Step 7: Submit your files
See the “What to Submit” section, below.
Specifications
You must implement the following functions. These functions are the public interface of the project. The public interface will be used to test your project. You can use private helper functions in your design of this project. They won't be called directly for testing.
- If the Course object is empty the function allocates proper memory with the default size DEFNUMSECTIONS.
- There must be at least one student and one test in the section to be inserted.
- The function does not insert a section with a duplicate ID.
- The function does not insert a section if there is no space in the Course object.
- If the insert operation is successful the function returns true.
- If the insert operation is not successful the function returns false.
- If the Course object is empty the function allocates proper memory with the default size DEFNUMSECTIONS.
- There must be at least one student and one test in the section to be inserted.
- The function does not insert a section with a duplicate ID.
- The function does not insert a section if there is no space in the Course object.
- The function does not insert a nullptr section, i.e. aSection parameter is nullptr.
- This function uses the pointer passed, it does not create a new pointer for aSection parameter.
- If the insert operation is successful the function returns true.
- If the insert operation is not successful the function returns false.
- There must be at least one student in a section.
- There must be at least one section in the course.
- If there is not enough information to perform the calculation the function returns zero.
- If the remove operation is successful the function returns true.
- If the remove operation is not successful the function returns false.
Additional Requirements
- The class declarations (Section and Course) in course.cpp may not be modified in any way. No additional libraries may be used.
- You muse use nullptr to set pointers to a default value. Do not use NULL or zero or any other value for this purpose.
- To generate random data you muse use the provided class Random in course.h.
- You are not allowed to use STL template containers in your project implementation.
- You are allowed to use STL template containers in your test functions.
- The class functions must be written in course.cpp; in particular, they must not be written “in-line.”
- Private helper functions may be added, but must be declared in the private section of the Section and Course classes. There is a comment indicating where private helper function declarations should be written.
- You should read through the coding standards for this course.
Testing
Important, points will be deducted for violating the following list:
- The sample driver.cpp file will not be accepted as a test file.
- The name of the test file must be mytest.cpp.
- The mytest.cpp file contains the Tester class, and the main function.
- The test cases must be implemented in the Tester class, and must be called in the main function.
- Every test function returns true or false to indicate the pass or fail.
- A dump() function is provided for debugging purposes, visual inspections will not be accepted as test cases.
You must write and submit a test program along with the implementation of your project. To test your project class, you implement your test functions in the Tester class. The Tester class resides in your test file. You name your test file mytest.cpp. It is strongly recommended that you read the testing guidelines before writing test cases. A sample test program including Tester class, and sample test functions are provided in driver.cpp. You add your Tester class, test functions and test cases to mytest.cpp. You must write a separate function for every test case.
Any program should be adequately tested. Adequate testing includes test functions for normal cases, edge cases and error cases if any applies. The following list presents some examples for this project:
- Trying to create a copy of an empty object would be an error case. This must be tested when using the overloaded assignment operator.
- Trying to create an object with one piece of data where the object can carry multiple pieces of data is an edge case.
- Trying to assign a well populated object to another well populated object is a normal case. This must be tested when using the overloaded assignment operator.
The following presents a non-exhaustive list of tests for this project. Please note, you need to test your work adequately.
- Test whether the constructor works correctly for a normal case.
- Test whether the constructor works correctly for an error case, e.g. it creates an empty object if the user tries to create an object with -5 grading items and -10 students.
- Test whether the copy constructor works correctly for a normal case. Here is a sample algorithm:
- Create a normal object,
- Create a copy of previous object using the copy constructor,
- Check whether m_section of original object and copy object point to different memory locations,
- Check whether the corresponding member variables of both objects carry the same values,
- Check whether all corresponding grades are equal in both objects.
- Test whether the copy constructor works correctly for an edge case, i.e. it copies an empty object correctly.
- Test whether the assignment operator works correctly for a normal case.
- Test whether the assignment operator works correctly for an edge case, i.e. it assigns an empty object correctly.
- Test whether studentAverage() is working correctly for a normal case.
- Test whether insertSection() is working correctly for a normal case.
- Test whether insertSection() is working correctly for an error case, i.e. the requested ID exists in the object (trying to insert a duplicate section ID).
- Test whether removeSection() is working correctly for a normal case.
What to Submit
You must submit the following files to the proj0 submit directory:
- course.h
- course.cpp
- mytest.cpp (Note: This file contains the declaration and implementation of your Tester class as well as all your test cases.)
If you followed the instructions in the Project Submission page to set up your directories, you can submit your code using the following command:
cp course.h course.cpp mytest.cpp ~/cs341proj/proj0/
Grading Rubric
For details of grading rubric please refer to Project Grading Policy